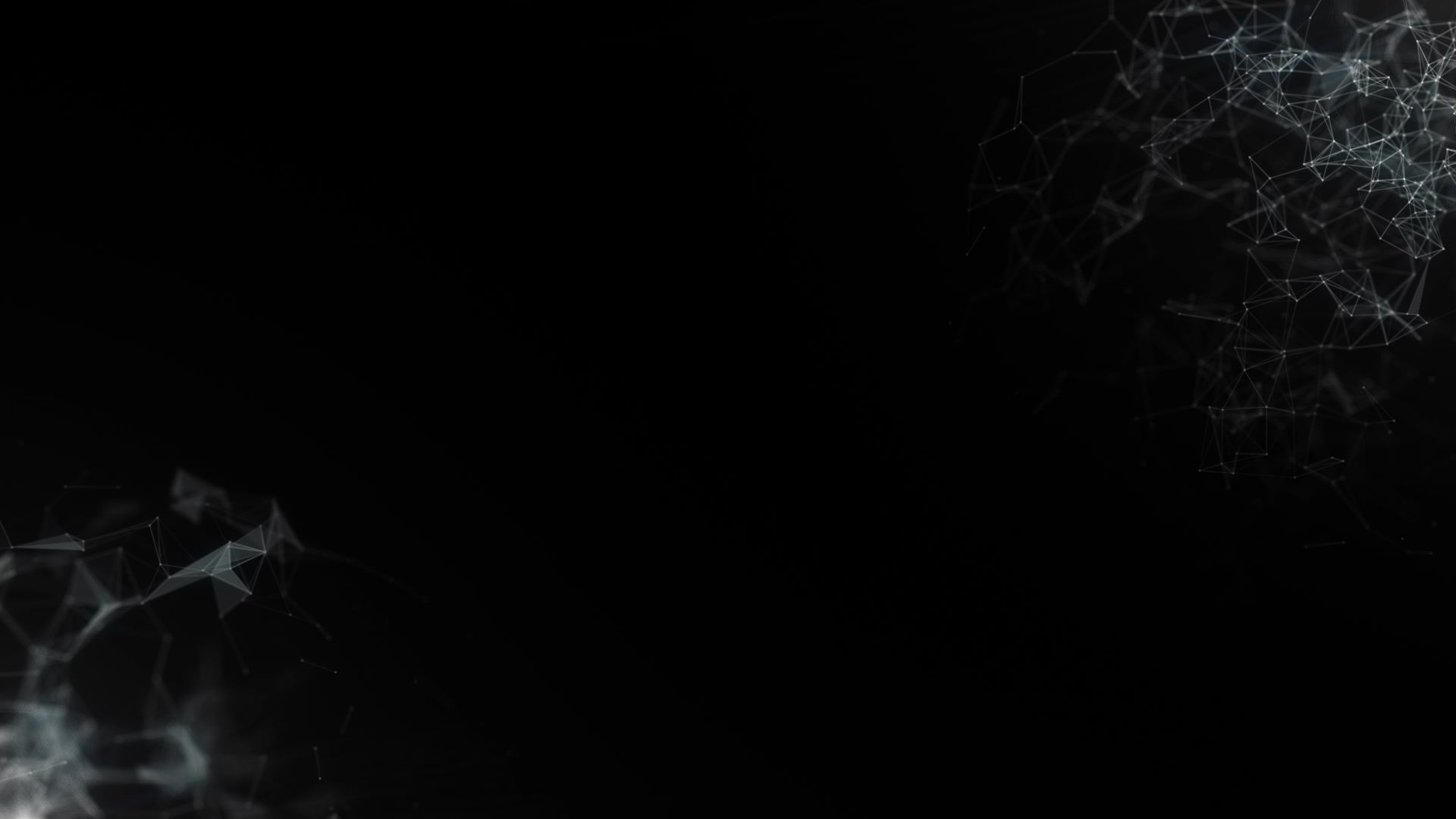
// imports
import greenfoot.*;
import java.awt.Color;
​
// fields
private Actor valueBar;
private int value = 3600;
private int maxValue = value;
// in constructor
valueBar = new SimpleActor();
updateValueDisplay();
addObject(valueBar, 80, 20);
​
// changing value
public void adjustValue(int amount)
{
value += amount;
if (value < 0) value = 0;
if (value > maxValue) value = maxValue;
updateValueDisplay();
}
​
// updating value display image
private void updateValueDisplay()
{
// fields for dimensions of color bar
int wide = 100; // width
int high = 12; // height
// create full color bar (at maximum value)
GreenfootImage fullImg = new GreenfootImage(wide, high);
fullImg.setColor(Color.GREEN);
fullImg.fill();
// create color bar (at value)
GreenfootImage colorBar = new GreenfootImage(wide, high);
int percentage = wide*value/maxValue;
colorBar.drawImage(fullImg, percentage-wide, 0);
// create actor image
GreenfootImage image = new GreenfootImage(wide+4, high+4);
image.setColor(Color.WHITE);
image.fill(); // the background
image.setColor(Color.BLACK);
image.drawRect(0, 0, wide+3, high+3); // the frame
image.drawImage(colorBar, 2, 2); // the color bar
valueBar.setImage(image);
}