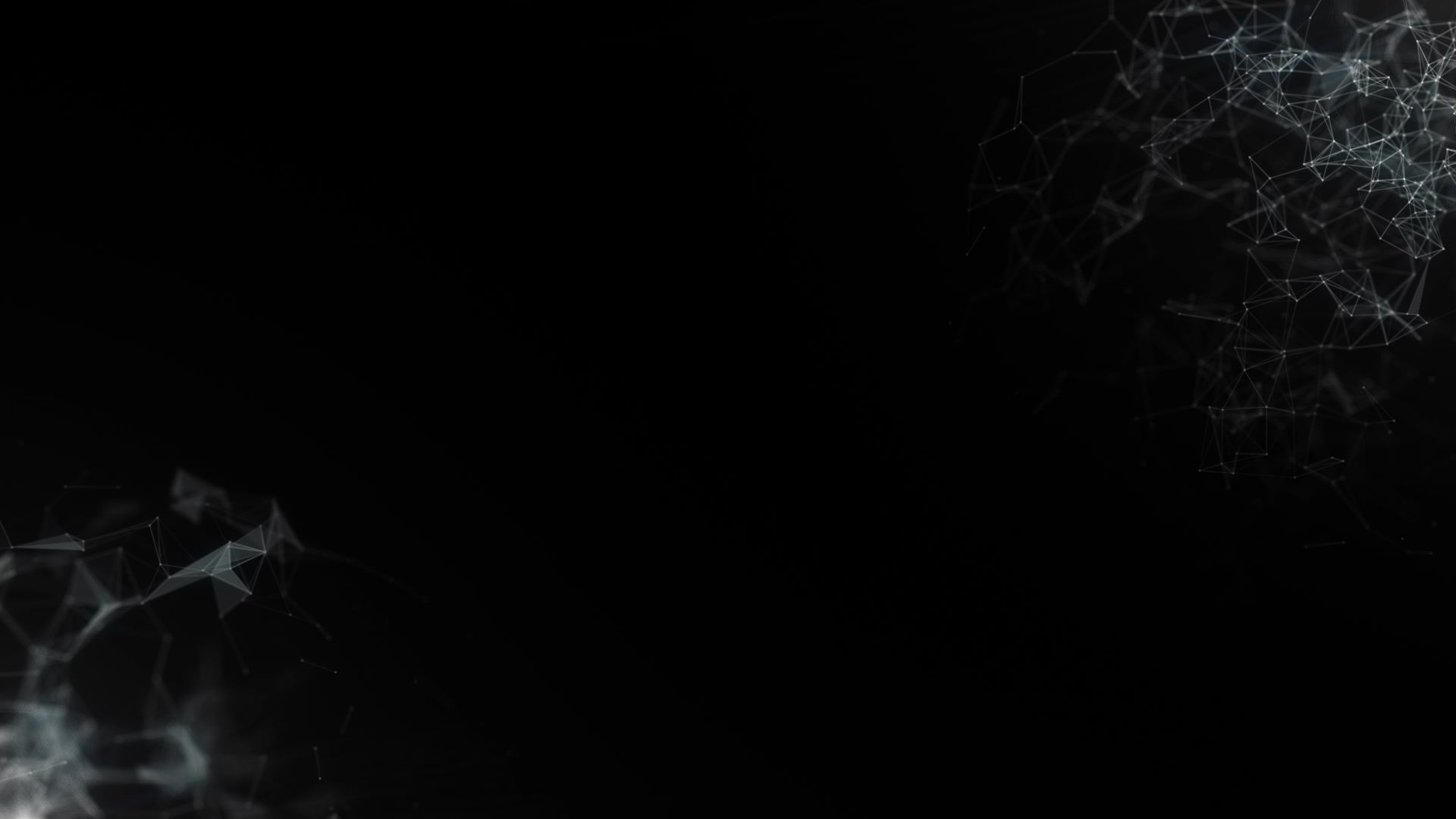
/**
* CLASS: MouseScrollWorld
*
* DESCRIPTION: creates a mouse dragging triggered scrolling world
*/
import greenfoot.*;
public class MouseScrollWorld extends World
{
// class fields
public static final int WIDE = 800; // world width (viewport)
public static final int HIGH = 600; // world height (viewport)
// instance fields
Scroller scroller; // reference to Scroller object
int dragX, dragY; // previous mouse position coordinates
// creates an unlimited scrolling world
public MouseScrollWorld()
{
super(WIDE, HIGH, 1, false); // create an unbounded world
scroller = new Scroller(this, new GreenfootImage("bg.png")); // Scroller obj
}
public void act()
{
scroll();
}
​
// performs mouse drag scrolling of world
private void scroll()
{
// mouse dragging check
if (Greenfoot.mouseDragged(this))
{
MouseInfo mInfo = Greenfoot.getMouseInfo();
// calculate change in position of mouse
int dsx = dragX-mInfo.getX();
int dsy = dragY-mInfo.getY();
// retain new mouse position
dragX = mInfo.getX();
dragY = mInfo.getY();
// scroll
scroller.scroll(dsx, dsy);
}
// mouse button press check (possible beginning of dragging)
if (Greenfoot.mousePressed(this))
{
MouseInfo mInfo = Greenfoot.getMouseInfo();
// retain current mouse position
dragX = mInfo.getX();
dragY = mInfo.getY();
}
}
}