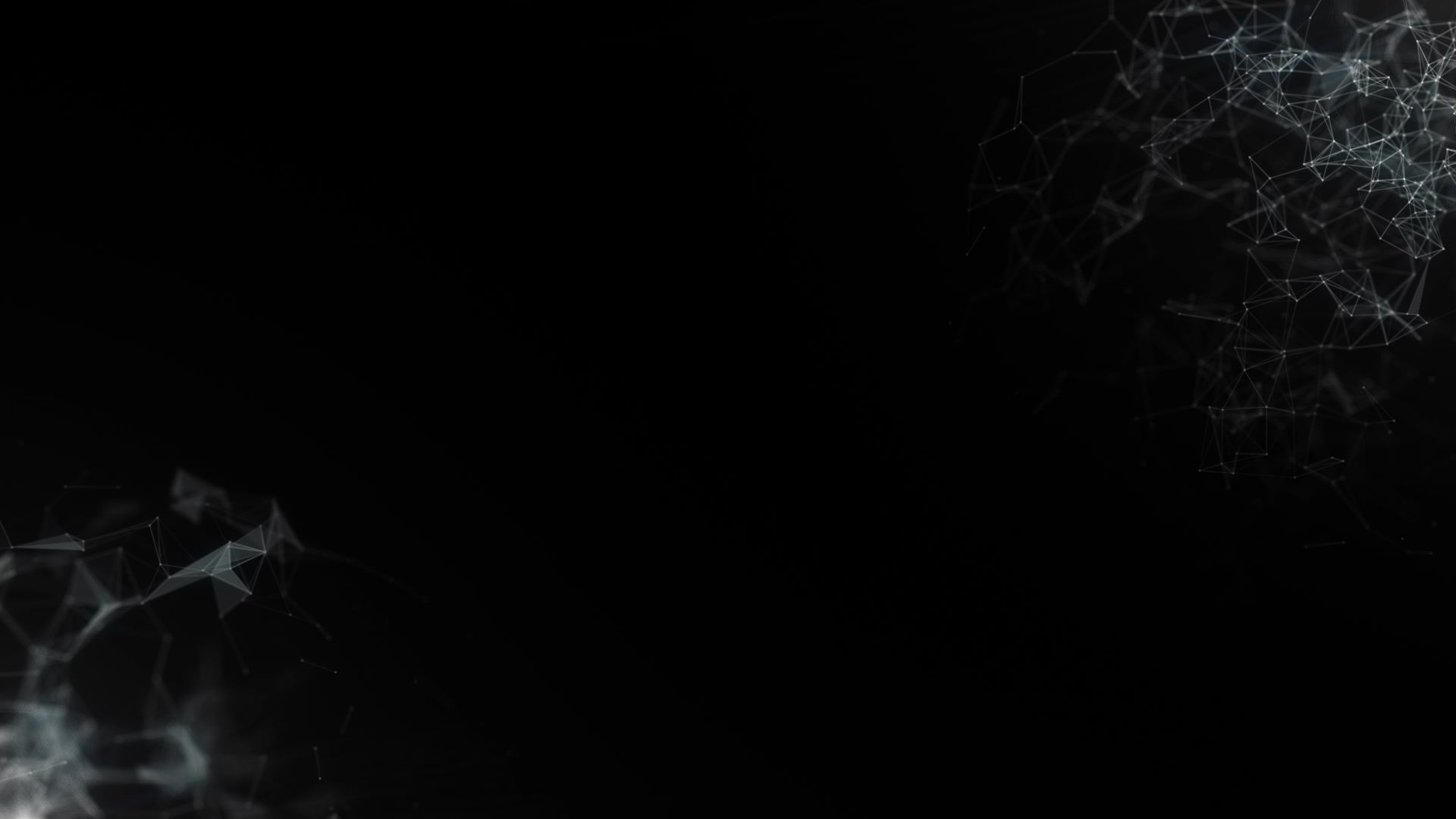
/**
* CLASS: KeyScrollWorld
*
* DESCRIPTION: a unlimited key controlled scrolling world
*/
​
import greenfoot.*;
public class KeyScrollWorld extends World
{
public static final int WIDE = 800; // world width (viewport)
public static final int HIGH = 600; // world height (viewport)
Scroller scroller; // the object that performs the scrolling
// creates a scrolling world
public KeyScrollWorld()
{
super(WIDE, HIGH, 1, false); // create an unbounded world
GreenfootImage image = new GreenfootImage("bg.png"); // the background image
scroller = new Scroller(this, image); // the Scroller object
}
​
public void act()
{
scroll();
}
// performs key controlled scrolling
private void scroll()
{
int rate = 2; // scroll speed
// determine scrolling offsets and scroll
int dsx = 0;
int dsy = 0;
if (Greenfoot.isKeyDown("right")) dsx++;
if (Greenfoot.isKeyDown("left")) dsx--;
if (Greenfoot.isKeyDown("down")) dsy++;
if (Greenfoot.isKeyDown("up")) dsy--;
scroller.scroll(dsx*rate, dsy*rate);
}
}