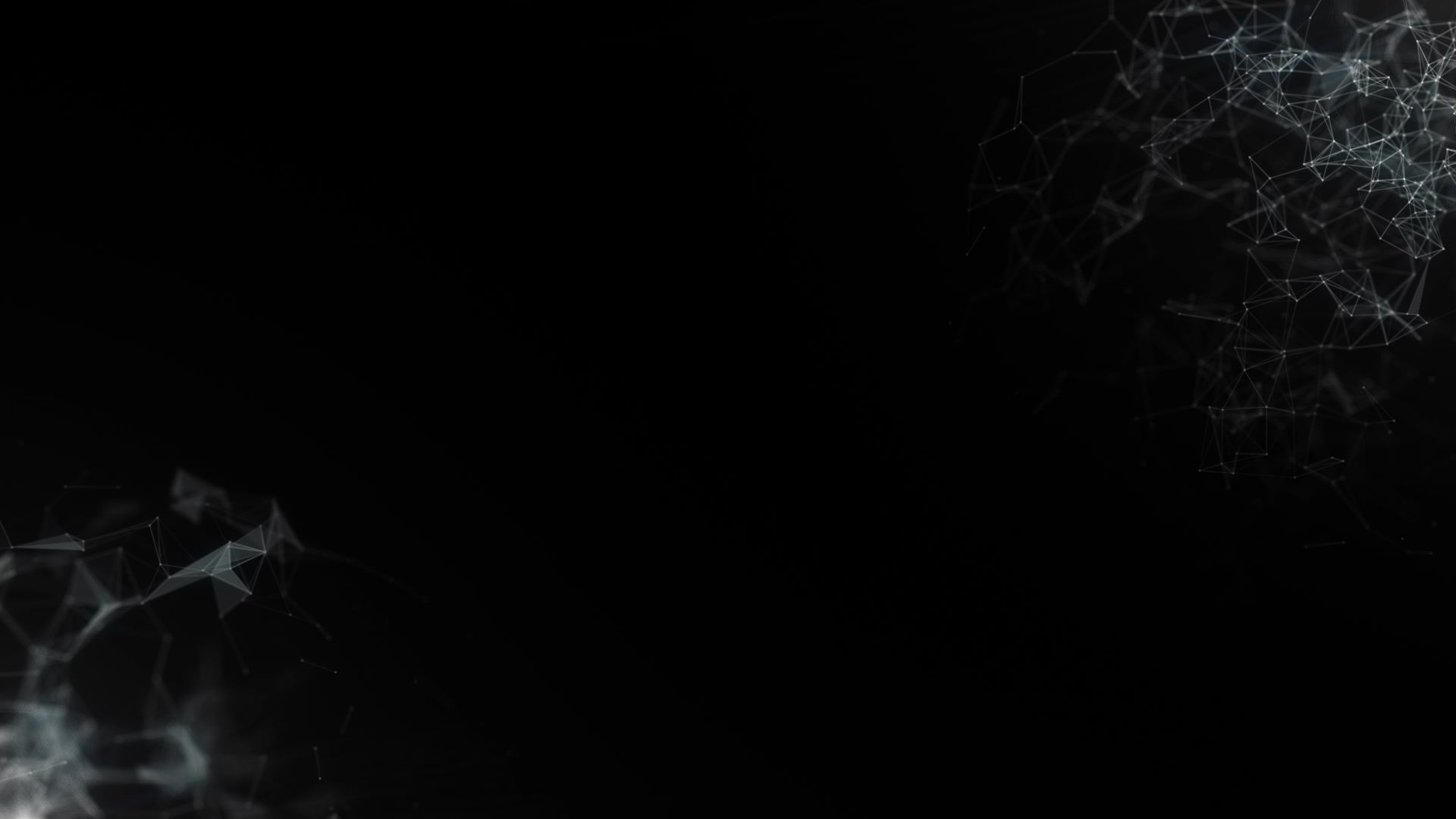
To build a basic Counter class out of a Text class, the first thing that needs to be done is to move the control field and the method to update the control value into the class. A method to return the current value is added also.
​
import greenfoot.*;
​
public class ValueDisplay extends Actor
{
private int value;
// updating image
private void updateImage(String text)
{
GreenfootImage image = new GreenfootImage(text, 24, null, null);
setImage(image);
}
// changing of value
public void adjustValue(int amount)
{
value += amount;
/* possible code to limit value goes here */
updateImage(""+value);
}
// returning the value
public int getValue()
{
return value;
}
}
Now, in order to label the value displayed, the class needs to retain the String value that is to prefix the value. So, a String field needs to be added with a method to change the prefix text. Then, the prefix and value can be used together when updating the image. A method to return the current prefix is also added.
​
import greenfoot.*;
​
public class Counter extends Actor
{
private String prefix = "";
private int value;
// updating image
private void updateImage()
{
String text = prefix+value;
GreenfootImage image = new GreenfootImage(text, 24, null, null);
setImage(image);
}
// changing of value
public void adjustValue(int amount)
{
value += amount;
updateImage();
}
// returning the value
public int getValue()
{
return value;
}
// changing of prefix
public void setPrefix(String text)
{
prefix = text;
if (prefix == null) prefix = "";
updateImage();
}
// returning the prefix
public String getPrefix()
{
return prefix;
}
}
With the above class, the following can be used when creating and adding the actor into the world:
​
// instance field
Counter timeDisplay;
// in constructor
timeDisplay = new Counter();
timeDisplay.setPrefix("Time remaining: ");
timeDisplay.adjustValue(3600);
addObject(timeDisplay, 80, 20);
It is wise to note that as a specialized class, you are creating more code and limiting what the Actor object created from the class could be used for. By maintaining the control value outside of the class of the actor that displays the representation of the value, any control value can be changed directly before updating the image of the actor. In other words, using a basic actor object, like a SimpleActor object, is much easier to code and more versatile to use than using a Text, Counter, Lives, HealthBar or any one of many other different specialized types of classes. I should probably make note that the only plus in creating these specialized classes is that, once created, they can easily be imported into other projects without any changes if coded properly. This saves a bit of time in building those project.