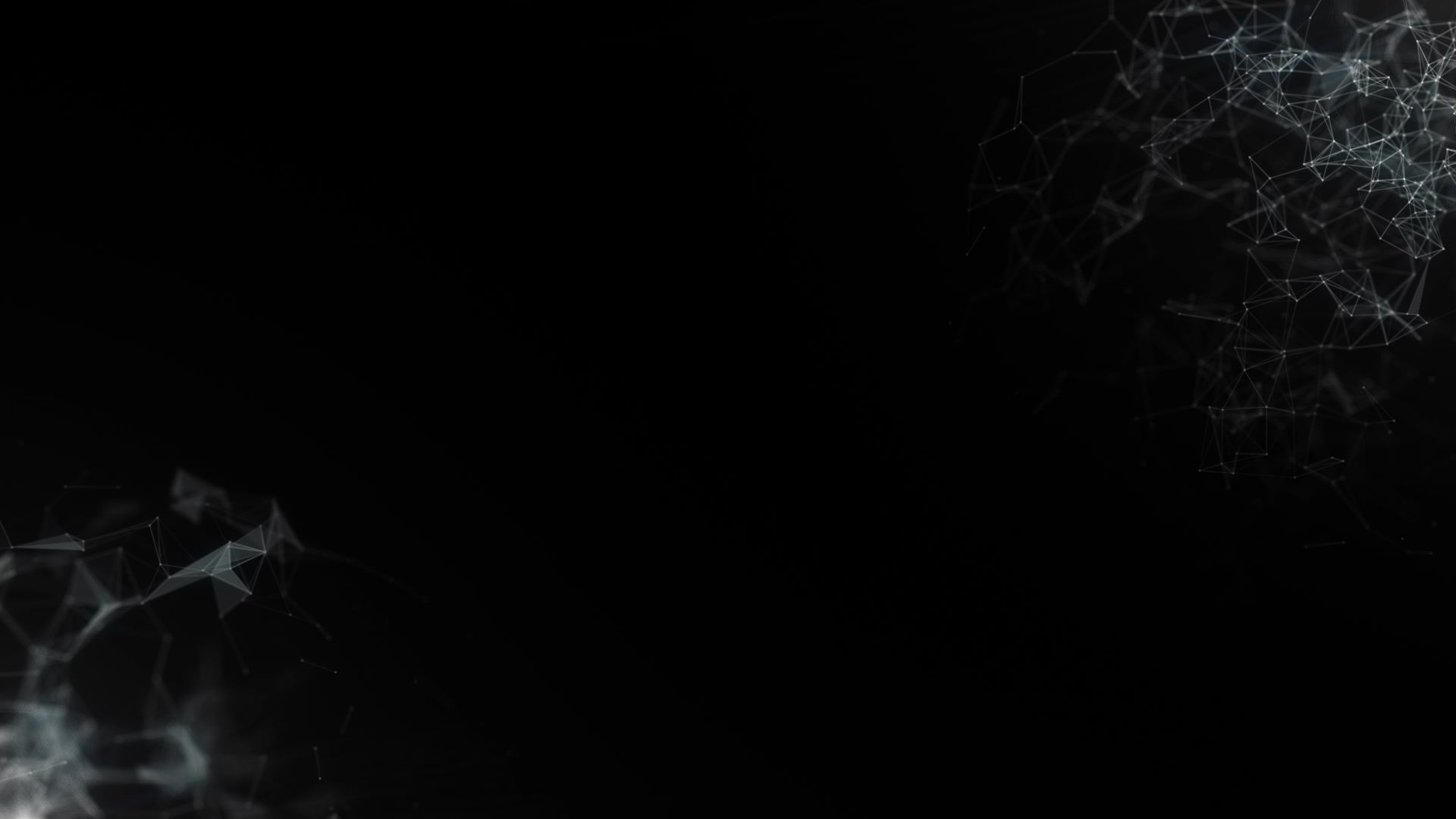
/**
* CLASS: ImageScrollWorld
*
* DESCRIPTION: creates a limited center screen actor scrolling world
*/
import greenfoot.*;
public class ImageScrollWorld extends World
{
public static final int WIDE = 800; // world width (viewport)
public static final int HIGH = 600; // world height (viewport)
Scroller scroller; // the object that performs the scrolling
Actor scrollActor; // an actor to stay in view
public ImageScrollWorld()
{
super(WIDE, HIGH, 1, false); // creates an unbounded world
GreenfootImage bg = new GreenfootImage("bg.png"); // the limiting image
int bgWide = bg.getWidth(); // scrolling image width
int bgHigh = bg.getHeight(); // scrolling image height
scroller = new Scroller(this, bg, bgWide, bgHigh); // the Scroller object
scrollActor = new Player(); // creates the actor to maintain view on
addObject(scrollActor, bgWide/2, bgHigh/2); // add actor to world (wherever)
scroll(); // sets initial background image and scrolls if needed
}
public void act()
{
if (scrollActor != null) scroll();
}
// attempts scrolling when actor is not in center of visible world
private void scroll()
{
// determine scrolling offsets and scroll
int dsx = scrollActor.getX()-WIDE/2; // horizontal offset from center screen
int dsy = scrollActor.getY()-HIGH/2; // vertical offset from center screen
scroller.scroll(dsx, dsy);
}
}
The following can be used to allow some actor movement before scrolling begins:
// attempts scrolling when actor is outside roaming limits
private void scroll()
{
// roaming limits of actor
int loX = 100;
int hiX = WIDE-100;
int loY = 50;
int hiY = HIGH-50;
// determine offsets and scroll
int dsx = 0, dsy = 0;
if (scrollActor.getX() < loX) dsx = scrollActor.getX()-loX;
if (scrollActor.getX() > hiX) dsx = scrollActor.getX()-hiX;
if (scrollActor.getY() < loY) dsy = scrollActor.getY()-loY;
if (scrollActor.getY() > hiY) dsy = scrollActor.getY()-hiY;
scroller.scroll(dsx, dsy);
}